All patches and comments are welcome. Please squash your changes to logical
commits before using git-format-patch and git-send-email to
patches@git.madduck.net.
If you'd read over the Git project's submission guidelines and adhered to them,
I'd be especially grateful.
1 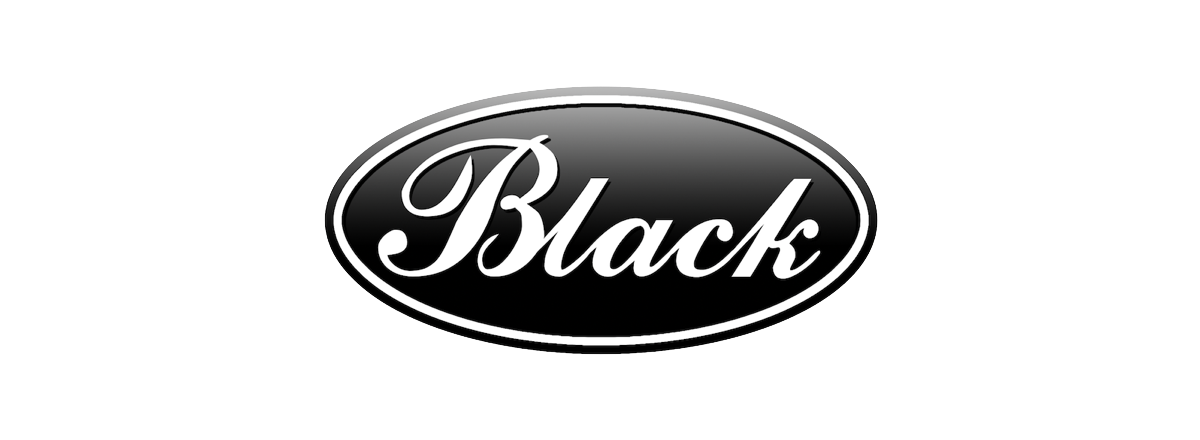
2 <h2 align="center">The Uncompromising Code Formatter</h2>
5 <a href="https://travis-ci.org/ambv/black"><img alt="Build Status" src="https://travis-ci.org/ambv/black.svg?branch=master"></a>
6 <a href="http://black.readthedocs.io/en/latest/?badge=latest"><img alt="Documentation Status" src="http://readthedocs.org/projects/black/badge/?version=latest"></a>
7 <a href="https://coveralls.io/github/ambv/black?branch=master"><img alt="Coverage Status" src="https://coveralls.io/repos/github/ambv/black/badge.svg?branch=master"></a>
8 <a href="https://github.com/ambv/black/blob/master/LICENSE"><img alt="License: MIT" src="http://black.readthedocs.io/en/latest/_static/license.svg"></a>
9 <a href="https://pypi.python.org/pypi/black"><img alt="PyPI" src="http://black.readthedocs.io/en/latest/_static/pypi.svg"></a>
10 <a href="https://github.com/ambv/black"><img alt="Code style: black" src="https://img.shields.io/badge/code%20style-black-000000.svg"></a>
13 > “Any color you like.”
16 *Black* is the uncompromising Python code formatter. By using it, you
17 agree to cease control over minutiae of hand-formatting. In return,
18 *Black* gives you speed, determinism, and freedom from `pycodestyle`
19 nagging about formatting. You will save time and mental energy for
20 more important matters.
22 Blackened code looks the same regardless of the project you're reading.
23 Formatting becomes transparent after a while and you can focus on the
26 *Black* makes code review faster by producing the smallest diffs
30 ## Installation and Usage
34 *Black* can be installed by running `pip install black`. It requires
35 Python 3.6.0+ to run but you can reformat Python 2 code with it, too.
40 To get started right away with sensible defaults:
43 black {source_file_or_directory}
46 ### Command line options
48 Black doesn't provide many options. You can list them by running
52 black [OPTIONS] [SRC]...
55 -l, --line-length INTEGER Where to wrap around. [default: 88]
56 --check Don't write back the files, just return the
57 status. Return code 0 means nothing would
58 change. Return code 1 means some files would be
59 reformatted. Return code 123 means there was an
61 --fast / --safe If --fast given, skip temporary sanity checks.
63 --version Show the version and exit.
64 --help Show this message and exit.
67 *Black* is a well-behaved Unix-style command-line tool:
68 * it does nothing if no sources are passed to it;
69 * it will read from standard input and write to standard output if `-`
70 is used as the filename;
71 * it only outputs messages to users on standard error;
72 * exits with code 0 unless an internal error occured (or `--check` was
76 ### NOTE: This is an early pre-release
78 *Black* can already successfully format itself and the standard library.
79 It also sports a decent test suite. However, it is still very new.
80 Things will probably be wonky for a while. This is made explicit by the
81 "Alpha" trove classifier, as well as by the "a" in the version number.
82 What this means for you is that **until the formatter becomes stable,
83 you should expect some formatting to change in the future**.
85 Also, as a temporary safety measure, *Black* will check that the
86 reformatted code still produces a valid AST that is equivalent to the
87 original. This slows it down. If you're feeling confident, use
91 ## The *Black* code style
93 *Black* reformats entire files in place. It is not configurable. It
94 doesn't take previous formatting into account. It doesn't reformat
95 blocks that start with `# fmt: off` and end with `# fmt: on`. It also
96 recognizes [YAPF](https://github.com/google/yapf)'s block comments to
97 the same effect, as a courtesy for straddling code.
100 ### How *Black* wraps lines
102 *Black* ignores previous formatting and applies uniform horizontal
103 and vertical whitespace to your code. The rules for horizontal
104 whitespace are pretty obvious and can be summarized as: do whatever
105 makes `pycodestyle` happy. The coding style used by *Black* can be
106 viewed as a strict subset of PEP 8.
108 As for vertical whitespace, *Black* tries to render one full expression
109 or simple statement per line. If this fits the allotted line length,
124 If not, *Black* will look at the contents of the first outer matching
125 brackets and put that in a separate indented line.
129 l = [[n for n in list_bosses()], [n for n in list_employees()]]
134 [n for n in list_bosses()], [n for n in list_employees()]
138 If that still doesn't fit the bill, it will decompose the internal
139 expression further using the same rule, indenting matching brackets
140 every time. If the contents of the matching brackets pair are
141 comma-separated (like an argument list, or a dict literal, and so on)
142 then *Black* will first try to keep them on the same line with the
143 matching brackets. If that doesn't work, it will put all of them in
148 def very_important_function(template: str, *variables, file: os.PathLike, debug: bool = False):
149 """Applies `variables` to the `template` and writes to `file`."""
150 with open(file, 'w') as f:
155 def very_important_function(
161 """Applies `variables` to the `template` and writes to `file`."""
162 with open(file, "w") as f:
166 You might have noticed that closing brackets are always dedented and
167 that a trailing comma is always added. Such formatting produces smaller
168 diffs; when you add or remove an element, it's always just one line.
169 Also, having the closing bracket dedented provides a clear delimiter
170 between two distinct sections of the code that otherwise share the same
171 indentation level (like the arguments list and the docstring in the
177 You probably noticed the peculiar default line length. *Black* defaults
178 to 88 characters per line, which happens to be 10% over 80. This number
179 was found to produce significantly shorter files than sticking with 80
180 (the most popular), or even 79 (used by the standard library). In
181 general, [90-ish seems like the wise choice](https://youtu.be/wf-BqAjZb8M?t=260).
183 If you're paid by the line of code you write, you can pass
184 `--line-length` with a lower number. *Black* will try to respect that.
185 However, sometimes it won't be able to without breaking other rules. In
186 those rare cases, auto-formatted code will exceed your allotted limit.
188 You can also increase it, but remember that people with sight disabilities
189 find it harder to work with line lengths exceeding 100 characters.
190 It also adversely affects side-by-side diff review on typical screen
191 resolutions. Long lines also make it harder to present code neatly
192 in documentation or talk slides.
194 If you're using Flake8, you can bump `max-line-length` to 88 and forget
195 about it. Alternatively, use [Bugbear](https://github.com/PyCQA/flake8-bugbear)'s
196 B950 warning instead of E501 and keep the max line length at 80 which
197 you are probably already using. You'd do it like this:
202 select = C,E,F,W,B,B950
206 You'll find *Black*'s own .flake8 config file is configured like this.
207 If you're curious about the reasoning behind B950, Bugbear's documentation
208 explains it. The tl;dr is "it's like highway speed limits, we won't
209 bother you if you overdo it by a few km/h".
214 *Black* avoids spurious vertical whitespace. This is in the spirit of
215 PEP 8 which says that in-function vertical whitespace should only be
216 used sparingly. One exception is control flow statements: *Black* will
217 always emit an extra empty line after ``return``, ``raise``, ``break``,
218 ``continue``, and ``yield``. This is to make changes in control flow
219 more prominent to readers of your code.
221 *Black* will allow single empty lines inside functions, and single and
222 double empty lines on module level left by the original editors, except
223 when they're within parenthesized expressions. Since such expressions
224 are always reformatted to fit minimal space, this whitespace is lost.
226 It will also insert proper spacing before and after function definitions.
227 It's one line before and after inner functions and two lines before and
228 after module-level functions. *Black* will put those empty lines also
229 between the function definition and any standalone comments that
230 immediately precede the given function. If you want to comment on the
231 entire function, use a docstring or put a leading comment in the function
237 *Black* will add trailing commas to expressions that are split
238 by comma where each element is on its own line. This includes function
241 Unnecessary trailing commas are removed if an expression fits in one
242 line. This makes it 1% more likely that your line won't exceed the
243 allotted line length limit. Moreover, in this scenario, if you added
244 another argument to your call, you'd probably fit it in the same line
245 anyway. That doesn't make diffs any larger.
247 One exception to removing trailing commas is tuple expressions with
248 just one element. In this case *Black* won't touch the single trailing
249 comma as this would unexpectedly change the underlying data type. Note
250 that this is also the case when commas are used while indexing. This is
251 a tuple in disguise: ```numpy_array[3, ]```.
253 One exception to adding trailing commas is function signatures
254 containing `*`, `*args`, or `**kwargs`. In this case a trailing comma
255 is only safe to use on Python 3.6. *Black* will detect if your file is
256 already 3.6+ only and use trailing commas in this situation. If you
257 wonder how it knows, it looks for f-strings and existing use of trailing
258 commas in function signatures that have stars in them. In other words,
259 if you'd like a trailing comma in this situation and *Black* didn't
260 recognize it was safe to do so, put it there manually and *Black* will
265 *Black* prefers double quotes (`"` and `"""`), but only if this does not
266 result in more escaping. It will remove escape sequences as necessary as
267 part of moving to the other type of quote. This applies to all kinds of
268 prefixed strings, including *raw-strings* (`r""`), *byte literals* (`b""`),
269 and *formatted strings* (`f""`). The approach above strikes a good balance
270 between consistency and legibility.
273 ## Editor integration
277 Use [proofit404/blacken](https://github.com/proofit404/blacken).
282 Commands and shortcuts:
284 * `,=` or `:Black` to format the entire file (ranges not supported);
285 * `:BlackUpgrade` to upgrade *Black* inside the virtualenv;
286 * `:BlackVersion` to get the current version of *Black* inside the
290 * `g:black_fast` (defaults to `0`)
291 * `g:black_linelength` (defaults to `88`)
292 * `g:black_virtualenv` (defaults to `~/.vim/black`)
294 To install, copy the plugin from [vim/plugin/black.vim](https://github.com/ambv/black/tree/master/vim/plugin/black.vim).
295 Let me know if this requires any changes to work with Vim 8's builtin
296 `packadd`, or Pathogen, or Vundle, and so on.
298 This plugin **requires Vim 7.0+ built with Python 3.6+ support**. It
299 needs Python 3.6 to be able to run *Black* inside the Vim process which
300 is much faster than calling an external command.
302 On first run, the plugin creates its own virtualenv using the right
303 Python version and automatically installs *Black*. You can upgrade it later
304 by calling `:BlackUpgrade` and restarting Vim.
306 If you need to do anything special to make your virtualenv work and
307 install *Black* (for example you want to run a version from master), just
308 create a virtualenv manually and point `g:black_virtualenv` to it.
309 The plugin will use it.
311 **How to get Vim with Python 3.6?**
312 On Ubuntu 17.10 Vim comes with Python 3.6 by default.
313 On macOS with HomeBrew run: `brew install vim --with-python3`.
314 When building Vim from source, use:
315 `./configure --enable-python3interp=yes`. There's many guides online how
319 ### Visual Studio Code
321 Use [joslarson.black-vscode](https://marketplace.visualstudio.com/items?itemName=joslarson.black-vscode).
326 Atom/Nuclide integration is planned by the author, others will
327 require external contributions.
329 Patches welcome! ✨ 🍰 ✨
331 Any tool that can pipe code through *Black* using its stdio mode (just
332 [use `-` as the file name](http://www.tldp.org/LDP/abs/html/special-chars.html#DASHREF2)).
333 The formatted code will be returned on stdout (unless `--check` was
334 passed). *Black* will still emit messages on stderr but that shouldn't
335 affect your use case.
337 This can be used for example with PyCharm's [File Watchers](https://www.jetbrains.com/help/pycharm/file-watchers.html).
342 **Dusty Phillips**, [writer](https://smile.amazon.com/s/ref=nb_sb_noss?url=search-alias%3Daps&field-keywords=dusty+phillips):
344 > Black is opinionated so you don't have to be.
346 **Hynek Schlawack**, [creator of `attrs`](http://www.attrs.org/), core
347 developer of Twisted and CPython:
349 > An auto-formatter that doesn't suck is all I want for Xmas!
351 **Carl Meyer**, [Django](https://www.djangoproject.com/) core developer:
353 > At least the name is good.
355 **Kenneth Reitz**, creator of [`requests`](http://python-requests.org/)
356 and [`pipenv`](https://docs.pipenv.org/):
358 > This vastly improves the formatting of our code. Thanks a ton!
363 Use the badge in your project's README.md:
366 [](https://github.com/ambv/black)
369 Looks like this: [](https://github.com/ambv/black)
377 ## Contributing to Black
379 In terms of inspiration, *Black* is about as configurable as *gofmt* and
380 *rustfmt* are. This is deliberate.
382 Bug reports and fixes are always welcome! However, before you suggest a
383 new feature or configuration knob, ask yourself why you want it. If it
384 enables better integration with some workflow, fixes an inconsistency,
385 speeds things up, and so on - go for it! On the other hand, if your
386 answer is "because I don't like a particular formatting" then you're not
387 ready to embrace *Black* yet. Such changes are unlikely to get accepted.
388 You can still try but prepare to be disappointed.
390 More details can be found in [CONTRIBUTING](CONTRIBUTING.md).
395 ### 18.3a5 (unreleased)
397 * add line breaks before all delimiters, except in cases like commas, to better
398 comply with PEP8 (#73)
400 * fixed handling of standalone comments within nested bracketed
401 expressions; Black will no longer produce super long lines or put all
402 standalone comments at the end of the expression (#22)
404 * fixed 18.3a4 regression: don't crash and burn on empty lines with
405 trailing whitespace (#80)
407 * when CTRL+C is pressed while formatting many files, Black no longer
408 freaks out with a flurry of asyncio-related exceptions
410 * only allow up to two empty lines on module level and only single empty
411 lines within functions (#74)
416 * `# fmt: off` and `# fmt: on` are implemented (#5)
418 * automatic detection of deprecated Python 2 forms of print statements
419 and exec statements in the formatted file (#49)
421 * use proper spaces for complex expressions in default values of typed
422 function arguments (#60)
424 * only return exit code 1 when --check is used (#50)
426 * don't remove single trailing commas from square bracket indexing
429 * don't omit whitespace if the previous factor leaf wasn't a math
432 * omit extra space in kwarg unpacking if it's the first argument (#46)
434 * omit extra space in [Sphinx auto-attribute comments](http://www.sphinx-doc.org/en/stable/ext/autodoc.html#directive-autoattribute)
440 * don't remove single empty lines outside of bracketed expressions
443 * added ability to pipe formatting from stdin to stdin (#25)
445 * restored ability to format code with legacy usage of `async` as
448 * even better handling of numpy-style array indexing (#33, again)
453 * changed positioning of binary operators to occur at beginning of lines
454 instead of at the end, following [a recent change to PEP8](https://github.com/python/peps/commit/c59c4376ad233a62ca4b3a6060c81368bd21e85b)
457 * ignore empty bracket pairs while splitting. This avoids very weirdly
458 looking formattings (#34, #35)
460 * remove a trailing comma if there is a single argument to a call
462 * if top level functions were separated by a comment, don't put four
463 empty lines after the upper function
465 * fixed unstable formatting of newlines with imports
467 * fixed unintentional folding of post scriptum standalone comments
468 into last statement if it was a simple statement (#18, #28)
470 * fixed missing space in numpy-style array indexing (#33)
472 * fixed spurious space after star-based unary expressions (#31)
479 * only put trailing commas in function signatures and calls if it's
480 safe to do so. If the file is Python 3.6+ it's always safe, otherwise
481 only safe if there are no `*args` or `**kwargs` used in the signature
484 * fixed invalid spacing of dots in relative imports (#6, #13)
486 * fixed invalid splitting after comma on unpacked variables in for-loops
489 * fixed spurious space in parenthesized set expressions (#7)
491 * fixed spurious space after opening parentheses and in default
494 * fixed spurious space after unary operators when the operand was
495 a complex expression (#15)
500 * first published version, Happy 🍰 Day 2018!
504 * date-versioned (see: https://calver.org/)
509 Glued together by [Łukasz Langa](mailto:lukasz@langa.pl).
511 Maintained with [Carol Willing](mailto:carolcode@willingconsulting.com)
512 and [Carl Meyer](mailto:carl@oddbird.net).
514 Multiple contributions by:
515 * [Artem Malyshev](mailto:proofit404@gmail.com)
516 * [Daniel M. Capella](mailto:polycitizen@gmail.com)
517 * [Eli Treuherz](mailto:eli.treuherz@cgi.com)
519 * [Mika Naylor](mailto:mail@autophagy.io)
520 * [Osaetin Daniel](mailto:osaetindaniel@gmail.com)