All patches and comments are welcome. Please squash your changes to logical
commits before using git-format-patch and git-send-email to
patches@git.madduck.net.
If you'd read over the Git project's submission guidelines and adhered to them,
I'd be especially grateful.
1 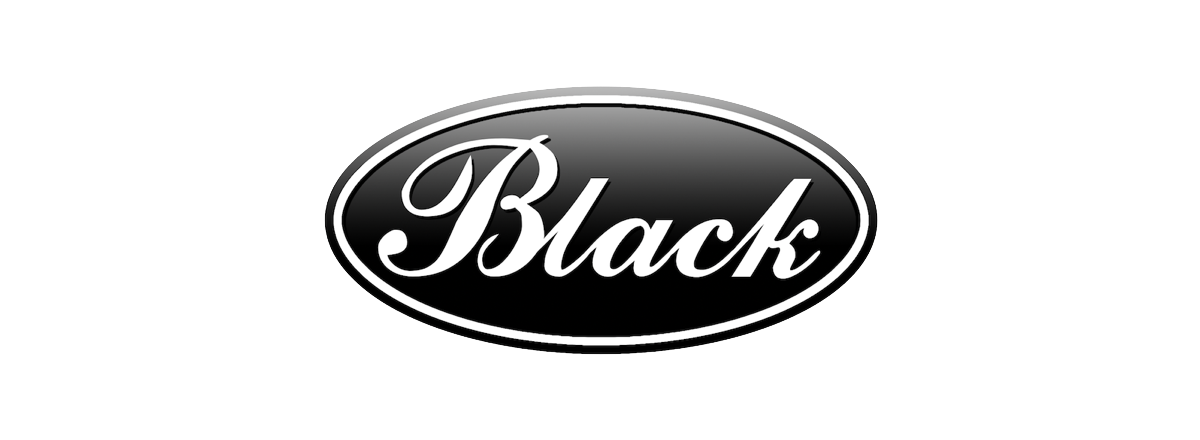
2 <h2 align="center">The Uncompromising Code Formatter</h2>
5 <a href="https://travis-ci.org/ambv/black"><img alt="Build Status" src="https://travis-ci.org/ambv/black.svg?branch=master"></a>
6 <a href="http://black.readthedocs.io/en/latest/?badge=latest"><img alt="Documentation Status" src="http://readthedocs.org/projects/black/badge/?version=latest"></a>
7 <a href="https://coveralls.io/github/ambv/black?branch=master"><img alt="Coverage Status" src="https://coveralls.io/repos/github/ambv/black/badge.svg?branch=master"></a>
8 <a href="https://github.com/ambv/black/blob/master/LICENSE"><img alt="License: MIT" src="http://black.readthedocs.io/en/latest/_static/license.svg"></a>
9 <a href="https://pypi.python.org/pypi/black"><img alt="PyPI" src="http://black.readthedocs.io/en/latest/_static/pypi.svg"></a>
10 <a href="https://github.com/ambv/black"><img alt="Code style: black" src="https://img.shields.io/badge/code%20style-black-000000.svg"></a>
13 > “Any color you like.”
16 *Black* is the uncompromising Python code formatter. By using it, you
17 agree to cede control over minutiae of hand-formatting. In return,
18 *Black* gives you speed, determinism, and freedom from `pycodestyle`
19 nagging about formatting. You will save time and mental energy for
20 more important matters.
22 Blackened code looks the same regardless of the project you're reading.
23 Formatting becomes transparent after a while and you can focus on the
26 *Black* makes code review faster by producing the smallest diffs
30 ## Installation and Usage
34 *Black* can be installed by running `pip install black`. It requires
35 Python 3.6.0+ to run but you can reformat Python 2 code with it, too.
40 To get started right away with sensible defaults:
43 black {source_file_or_directory}
46 ### Command line options
48 Black doesn't provide many options. You can list them by running
52 black [OPTIONS] [SRC]...
55 -l, --line-length INTEGER Where to wrap around. [default: 88]
56 --check Don't write the files back, just return the
57 status. Return code 0 means nothing would
58 change. Return code 1 means some files would be
59 reformatted. Return code 123 means there was an
61 --diff Don't write the files back, just output a diff
62 for each file on stdout.
63 --fast / --safe If --fast given, skip temporary sanity checks.
65 -q, --quiet Don't emit non-error messages to stderr. Errors
66 are still emitted, silence those with
68 --version Show the version and exit.
69 --help Show this message and exit.
72 *Black* is a well-behaved Unix-style command-line tool:
73 * it does nothing if no sources are passed to it;
74 * it will read from standard input and write to standard output if `-`
75 is used as the filename;
76 * it only outputs messages to users on standard error;
77 * exits with code 0 unless an internal error occured (or `--check` was
81 ### NOTE: This is an early pre-release
83 *Black* can already successfully format itself and the standard library.
84 It also sports a decent test suite. However, it is still very new.
85 Things will probably be wonky for a while. This is made explicit by the
86 "Alpha" trove classifier, as well as by the "a" in the version number.
87 What this means for you is that **until the formatter becomes stable,
88 you should expect some formatting to change in the future**.
90 Also, as a temporary safety measure, *Black* will check that the
91 reformatted code still produces a valid AST that is equivalent to the
92 original. This slows it down. If you're feeling confident, use
96 ## The *Black* code style
98 *Black* reformats entire files in place. It is not configurable. It
99 doesn't take previous formatting into account. It doesn't reformat
100 blocks that start with `# fmt: off` and end with `# fmt: on`. It also
101 recognizes [YAPF](https://github.com/google/yapf)'s block comments to
102 the same effect, as a courtesy for straddling code.
105 ### How *Black* wraps lines
107 *Black* ignores previous formatting and applies uniform horizontal
108 and vertical whitespace to your code. The rules for horizontal
109 whitespace are pretty obvious and can be summarized as: do whatever
110 makes `pycodestyle` happy. The coding style used by *Black* can be
111 viewed as a strict subset of PEP 8.
113 As for vertical whitespace, *Black* tries to render one full expression
114 or simple statement per line. If this fits the allotted line length,
129 If not, *Black* will look at the contents of the first outer matching
130 brackets and put that in a separate indented line.
134 l = [[n for n in list_bosses()], [n for n in list_employees()]]
139 [n for n in list_bosses()], [n for n in list_employees()]
143 If that still doesn't fit the bill, it will decompose the internal
144 expression further using the same rule, indenting matching brackets
145 every time. If the contents of the matching brackets pair are
146 comma-separated (like an argument list, or a dict literal, and so on)
147 then *Black* will first try to keep them on the same line with the
148 matching brackets. If that doesn't work, it will put all of them in
153 def very_important_function(template: str, *variables, file: os.PathLike, debug: bool = False):
154 """Applies `variables` to the `template` and writes to `file`."""
155 with open(file, 'w') as f:
160 def very_important_function(
166 """Applies `variables` to the `template` and writes to `file`."""
167 with open(file, "w") as f:
171 You might have noticed that closing brackets are always dedented and
172 that a trailing comma is always added. Such formatting produces smaller
173 diffs; when you add or remove an element, it's always just one line.
174 Also, having the closing bracket dedented provides a clear delimiter
175 between two distinct sections of the code that otherwise share the same
176 indentation level (like the arguments list and the docstring in the
179 If a line of "from" imports cannot fit in the allotted length, it's always split
180 into one per line. Imports tend to change often and this minimizes diffs, as well
181 as enables readers of code to easily find which commit introduced a particular
182 import. This exception also makes *Black* compatible with
183 [isort](https://pypi.org/p/isort/). Use `multi_line_output=3` and
184 `include_trailing_comma=True` in your isort config.
189 You probably noticed the peculiar default line length. *Black* defaults
190 to 88 characters per line, which happens to be 10% over 80. This number
191 was found to produce significantly shorter files than sticking with 80
192 (the most popular), or even 79 (used by the standard library). In
193 general, [90-ish seems like the wise choice](https://youtu.be/wf-BqAjZb8M?t=260).
195 If you're paid by the line of code you write, you can pass
196 `--line-length` with a lower number. *Black* will try to respect that.
197 However, sometimes it won't be able to without breaking other rules. In
198 those rare cases, auto-formatted code will exceed your allotted limit.
200 You can also increase it, but remember that people with sight disabilities
201 find it harder to work with line lengths exceeding 100 characters.
202 It also adversely affects side-by-side diff review on typical screen
203 resolutions. Long lines also make it harder to present code neatly
204 in documentation or talk slides.
206 If you're using Flake8, you can bump `max-line-length` to 88 and forget
207 about it. Alternatively, use [Bugbear](https://github.com/PyCQA/flake8-bugbear)'s
208 B950 warning instead of E501 and keep the max line length at 80 which
209 you are probably already using. You'd do it like this:
214 select = C,E,F,W,B,B950
218 You'll find *Black*'s own .flake8 config file is configured like this.
219 If you're curious about the reasoning behind B950, Bugbear's documentation
220 explains it. The tl;dr is "it's like highway speed limits, we won't
221 bother you if you overdo it by a few km/h".
226 *Black* avoids spurious vertical whitespace. This is in the spirit of
227 PEP 8 which says that in-function vertical whitespace should only be
230 *Black* will allow single empty lines inside functions, and single and
231 double empty lines on module level left by the original editors, except
232 when they're within parenthesized expressions. Since such expressions
233 are always reformatted to fit minimal space, this whitespace is lost.
235 It will also insert proper spacing before and after function definitions.
236 It's one line before and after inner functions and two lines before and
237 after module-level functions. *Black* will not put empty lines between
238 function/class definitions and standalone comments that immediately precede
239 the given function/class.
244 *Black* will add trailing commas to expressions that are split
245 by comma where each element is on its own line. This includes function
248 Unnecessary trailing commas are removed if an expression fits in one
249 line. This makes it 1% more likely that your line won't exceed the
250 allotted line length limit. Moreover, in this scenario, if you added
251 another argument to your call, you'd probably fit it in the same line
252 anyway. That doesn't make diffs any larger.
254 One exception to removing trailing commas is tuple expressions with
255 just one element. In this case *Black* won't touch the single trailing
256 comma as this would unexpectedly change the underlying data type. Note
257 that this is also the case when commas are used while indexing. This is
258 a tuple in disguise: ```numpy_array[3, ]```.
260 One exception to adding trailing commas is function signatures
261 containing `*`, `*args`, or `**kwargs`. In this case a trailing comma
262 is only safe to use on Python 3.6. *Black* will detect if your file is
263 already 3.6+ only and use trailing commas in this situation. If you
264 wonder how it knows, it looks for f-strings and existing use of trailing
265 commas in function signatures that have stars in them. In other words,
266 if you'd like a trailing comma in this situation and *Black* didn't
267 recognize it was safe to do so, put it there manually and *Black* will
272 *Black* prefers double quotes (`"` and `"""`) over single quotes (`'`
273 and `'''`). It will replace the latter with the former as long as it
274 does not result in more backslash escapes than before.
276 The main reason to standardize on a single form of quotes is aesthetics.
277 Having one kind of quotes everywhere reduces reader distraction.
278 It will also enable a future version of *Black* to merge consecutive
279 string literals that ended up on the same line (see
280 [#26](https://github.com/ambv/black/issues/26) for details).
282 Why settle on double quotes? They anticipate apostrophes in English
283 text. They match the docstring standard described in PEP 257. An
284 empty string in double quotes (`""`) is impossible to confuse with
285 a one double-quote regardless of fonts and syntax highlighting used.
286 On top of this, double quotes for strings are consistent with C which
287 Python interacts a lot with.
289 On certain keyboard layouts like US English, typing single quotes is
290 a bit easier than double quotes. The latter requires use of the Shift
291 key. My recommendation here is to keep using whatever is faster to type
292 and let *Black* handle the transformation.
294 ### Line Breaks & Binary Operators
296 *Black* will break a line before a binary operator when splitting a block
297 of code over multiple lines. This is so that *Black* is compliant with the
298 recent changes in the [PEP 8](https://www.python.org/dev/peps/pep-0008/#should-a-line-break-before-or-after-a-binary-operator)
299 style guide, which emphasizes that this approach improves readability.
301 This behaviour may raise ``W503 line break before binary operator`` warnings in
302 style guide enforcement tools like Flake8. Since ``W503`` is not PEP 8 compliant,
303 you should tell Flake8 to ignore these warnings.
307 PEP 8 [recommends](https://www.python.org/dev/peps/pep-0008/#whitespace-in-expressions-and-statements)
308 to treat ``:`` in slices as a binary operator with the lowest priority, and to
309 leave an equal amount of space on either side, except if a parameter is omitted
310 (e.g. ``ham[1 + 1 :]``). It also states that for extended slices, both ``:``
311 operators have to have the same amount of spacing, except if a parameter is
312 omitted (``ham[1 + 1 ::]``). *Black* enforces these rules consistently.
314 This behaviour may raise ``E203 whitespace before ':'`` warnings in style guide
315 enforcement tools like Flake8. Since ``E203`` is not PEP 8 compliant, you should
316 tell Flake8 to ignore these warnings.
320 Some parentheses are optional in the Python grammar. Any expression can
321 be wrapped in a pair of parentheses to form an atom. There are a few
326 - `for (...) in (...):`
327 - `assert (...), (...)`
328 - `from X import (...)`
330 In those cases, parentheses are removed when the entire statement fits
331 in one line, or if the inner expression doesn't have any delimiters to
332 further split on. Otherwise, the parentheses are always added.
335 ## Editor integration
339 Use [proofit404/blacken](https://github.com/proofit404/blacken).
348 2. Locate your `black` installation folder.
350 On MacOS / Linux / BSD:
353 /usr/local/bin/black # possible location
358 %LocalAppData%\Programs\Python\Python36-32\Scripts\black.exe # possible location
360 3. Open External tools in PyCharm with `File -> Settings -> Tools -> External Tools`.
362 4. Click the + icon to add a new external tool with the following values:
364 - Description: Black is the uncompromising Python code formatter.
365 - Program: <install_location_from_step_2>
366 - Arguments: $FilePath$
368 5. Format the currently opened file by selecting `Tools -> External Tools -> black`.
369 - Alternatively, you can set a keyboard shortcut by navigating to `Preferences -> Keymap`.
374 Commands and shortcuts:
376 * `,=` or `:Black` to format the entire file (ranges not supported);
377 * `:BlackUpgrade` to upgrade *Black* inside the virtualenv;
378 * `:BlackVersion` to get the current version of *Black* inside the
382 * `g:black_fast` (defaults to `0`)
383 * `g:black_linelength` (defaults to `88`)
384 * `g:black_virtualenv` (defaults to `~/.vim/black`)
386 To install with [vim-plug](https://github.com/junegunn/vim-plug):
392 or with [Vundle](https://github.com/VundleVim/Vundle.vim):
398 or you can copy the plugin from [plugin/black.vim](https://github.com/ambv/black/tree/master/plugin/black.vim).
399 Let me know if this requires any changes to work with Vim 8's builtin
400 `packadd`, or Pathogen, and so on.
402 This plugin **requires Vim 7.0+ built with Python 3.6+ support**. It
403 needs Python 3.6 to be able to run *Black* inside the Vim process which
404 is much faster than calling an external command.
406 On first run, the plugin creates its own virtualenv using the right
407 Python version and automatically installs *Black*. You can upgrade it later
408 by calling `:BlackUpgrade` and restarting Vim.
410 If you need to do anything special to make your virtualenv work and
411 install *Black* (for example you want to run a version from master), just
412 create a virtualenv manually and point `g:black_virtualenv` to it.
413 The plugin will use it.
415 **How to get Vim with Python 3.6?**
416 On Ubuntu 17.10 Vim comes with Python 3.6 by default.
417 On macOS with HomeBrew run: `brew install vim --with-python3`.
418 When building Vim from source, use:
419 `./configure --enable-python3interp=yes`. There's many guides online how
423 ### Visual Studio Code
425 Use [joslarson.black-vscode](https://marketplace.visualstudio.com/items?itemName=joslarson.black-vscode).
429 Use [sublack plugin](https://github.com/jgirardet/sublack).
433 Atom/Nuclide integration is planned by the author, others will
434 require external contributions.
436 Patches welcome! ✨ 🍰 ✨
438 Any tool that can pipe code through *Black* using its stdio mode (just
439 [use `-` as the file name](http://www.tldp.org/LDP/abs/html/special-chars.html#DASHREF2)).
440 The formatted code will be returned on stdout (unless `--check` was
441 passed). *Black* will still emit messages on stderr but that shouldn't
442 affect your use case.
444 This can be used for example with PyCharm's [File Watchers](https://www.jetbrains.com/help/pycharm/file-watchers.html).
447 ## Version control integration
449 Use [pre-commit](https://pre-commit.com/). Once you [have it
450 installed](https://pre-commit.com/#install), add this to the
451 `.pre-commit-config.yaml` in your repository:
454 - repo: https://github.com/ambv/black
458 args: [--line-length=88, --safe]
459 python_version: python3.6
461 Then run `pre-commit install` and you're ready to go.
463 `args` in the above config is optional but shows you how you can change
464 the line length if you really need to. If you're already using Python
465 3.7, switch the `python_version` accordingly. Finally, `stable` is a tag
466 that is pinned to the latest release on PyPI. If you'd rather run on
467 master, this is also an option.
470 ## Ignoring non-modified files
472 *Black* remembers files it already formatted, unless the `--diff` flag is used or
473 code is passed via standard input. This information is stored per-user. The exact
474 location of the file depends on the black version and the system on which black
475 is run. The file is non-portable. The standard location on common operating systems
478 * Windows: `C:\\Users\<username>\AppData\Local\black\black\Cache\<version>\cache.<line-length>.pickle`
479 * macOS: `/Users/<username>/Library/Caches/black/<version>/cache.<line-length>.pickle`
480 * Linux: `/home/<username>/.cache/black/<version>/cache.<line-length>.pickle`
485 **Dusty Phillips**, [writer](https://smile.amazon.com/s/ref=nb_sb_noss?url=search-alias%3Daps&field-keywords=dusty+phillips):
487 > Black is opinionated so you don't have to be.
489 **Hynek Schlawack**, [creator of `attrs`](http://www.attrs.org/), core
490 developer of Twisted and CPython:
492 > An auto-formatter that doesn't suck is all I want for Xmas!
494 **Carl Meyer**, [Django](https://www.djangoproject.com/) core developer:
496 > At least the name is good.
498 **Kenneth Reitz**, creator of [`requests`](http://python-requests.org/)
499 and [`pipenv`](https://docs.pipenv.org/):
501 > This vastly improves the formatting of our code. Thanks a ton!
506 Use the badge in your project's README.md:
509 [](https://github.com/ambv/black)
512 Looks like this: [](https://github.com/ambv/black)
520 ## Contributing to Black
522 In terms of inspiration, *Black* is about as configurable as *gofmt*.
525 Bug reports and fixes are always welcome! However, before you suggest a
526 new feature or configuration knob, ask yourself why you want it. If it
527 enables better integration with some workflow, fixes an inconsistency,
528 speeds things up, and so on - go for it! On the other hand, if your
529 answer is "because I don't like a particular formatting" then you're not
530 ready to embrace *Black* yet. Such changes are unlikely to get accepted.
531 You can still try but prepare to be disappointed.
533 More details can be found in [CONTRIBUTING](CONTRIBUTING.md).
540 * don't populate the cache on `--check` (#175)
545 * added a "cache"; files already reformatted that haven't changed on disk
546 won't be reformatted again (#109)
548 * `--check` and `--diff` are no longer mutually exclusive (#149)
550 * generalized star expression handling, including double stars; this
551 fixes multiplication making expressions "unsafe" for trailing commas (#132)
553 * Black no longer enforces putting empty lines behind control flow statements
556 * Black now splits imports like "Mode 3 + trailing comma" of isort (#127)
558 * fixed comment indentation when a standalone comment closes a block (#16, #32)
560 * fixed standalone comments receiving extra empty lines if immediately preceding
561 a class, def, or decorator (#56, #154)
563 * fixed `--diff` not showing entire path (#130)
565 * fixed parsing of complex expressions after star and double stars in
568 * fixed invalid splitting on comma in lambda arguments (#133)
570 * fixed missing splits of ternary expressions (#141)
575 * fixed parsing of unaligned standalone comments (#99, #112)
577 * fixed placement of dictionary unpacking inside dictionary literals (#111)
579 * Vim plugin now works on Windows, too
581 * fixed unstable formatting when encountering unnecessarily escaped quotes
587 * added `--quiet` (#78)
589 * added automatic parentheses management (#4)
591 * added [pre-commit](https://pre-commit.com) integration (#103, #104)
593 * fixed reporting on `--check` with multiple files (#101, #102)
595 * fixed removing backslash escapes from raw strings (#100, #105)
600 * added `--diff` (#87)
602 * add line breaks before all delimiters, except in cases like commas, to
603 better comply with PEP 8 (#73)
605 * standardize string literals to use double quotes (almost) everywhere
608 * fixed handling of standalone comments within nested bracketed
609 expressions; Black will no longer produce super long lines or put all
610 standalone comments at the end of the expression (#22)
612 * fixed 18.3a4 regression: don't crash and burn on empty lines with
613 trailing whitespace (#80)
615 * fixed 18.3a4 regression: `# yapf: disable` usage as trailing comment
616 would cause Black to not emit the rest of the file (#95)
618 * when CTRL+C is pressed while formatting many files, Black no longer
619 freaks out with a flurry of asyncio-related exceptions
621 * only allow up to two empty lines on module level and only single empty
622 lines within functions (#74)
627 * `# fmt: off` and `# fmt: on` are implemented (#5)
629 * automatic detection of deprecated Python 2 forms of print statements
630 and exec statements in the formatted file (#49)
632 * use proper spaces for complex expressions in default values of typed
633 function arguments (#60)
635 * only return exit code 1 when --check is used (#50)
637 * don't remove single trailing commas from square bracket indexing
640 * don't omit whitespace if the previous factor leaf wasn't a math
643 * omit extra space in kwarg unpacking if it's the first argument (#46)
645 * omit extra space in [Sphinx auto-attribute comments](http://www.sphinx-doc.org/en/stable/ext/autodoc.html#directive-autoattribute)
651 * don't remove single empty lines outside of bracketed expressions
654 * added ability to pipe formatting from stdin to stdin (#25)
656 * restored ability to format code with legacy usage of `async` as
659 * even better handling of numpy-style array indexing (#33, again)
664 * changed positioning of binary operators to occur at beginning of lines
665 instead of at the end, following [a recent change to PEP8](https://github.com/python/peps/commit/c59c4376ad233a62ca4b3a6060c81368bd21e85b)
668 * ignore empty bracket pairs while splitting. This avoids very weirdly
669 looking formattings (#34, #35)
671 * remove a trailing comma if there is a single argument to a call
673 * if top level functions were separated by a comment, don't put four
674 empty lines after the upper function
676 * fixed unstable formatting of newlines with imports
678 * fixed unintentional folding of post scriptum standalone comments
679 into last statement if it was a simple statement (#18, #28)
681 * fixed missing space in numpy-style array indexing (#33)
683 * fixed spurious space after star-based unary expressions (#31)
690 * only put trailing commas in function signatures and calls if it's
691 safe to do so. If the file is Python 3.6+ it's always safe, otherwise
692 only safe if there are no `*args` or `**kwargs` used in the signature
695 * fixed invalid spacing of dots in relative imports (#6, #13)
697 * fixed invalid splitting after comma on unpacked variables in for-loops
700 * fixed spurious space in parenthesized set expressions (#7)
702 * fixed spurious space after opening parentheses and in default
705 * fixed spurious space after unary operators when the operand was
706 a complex expression (#15)
711 * first published version, Happy 🍰 Day 2018!
715 * date-versioned (see: https://calver.org/)
720 Glued together by [Łukasz Langa](mailto:lukasz@langa.pl).
722 Maintained with [Carol Willing](mailto:carolcode@willingconsulting.com),
723 [Carl Meyer](mailto:carl@oddbird.net),
724 [Mika Naylor](mailto:mail@autophagy.io), and
725 [Zsolt Dollenstein](mailto:zsol.zsol@gmail.com).
727 Multiple contributions by:
728 * [Anthony Sottile](mailto:asottile@umich.edu)
729 * [Artem Malyshev](mailto:proofit404@gmail.com)
730 * [Daniel M. Capella](mailto:polycitizen@gmail.com)
731 * [Eli Treuherz](mailto:eli.treuherz@cgi.com)
733 * [Ivan Katanić](mailto:ivan.katanic@gmail.com)
734 * [Jonas Obrist](mailto:ojiidotch@gmail.com)
735 * [Osaetin Daniel](mailto:osaetindaniel@gmail.com)
736 * [Vishwas B Sharma](mailto:sharma.vishwas88@gmail.com)