All patches and comments are welcome. Please squash your changes to logical
commits before using git-format-patch and git-send-email to
patches@git.madduck.net.
If you'd read over the Git project's submission guidelines and adhered to them,
I'd be especially grateful.
1 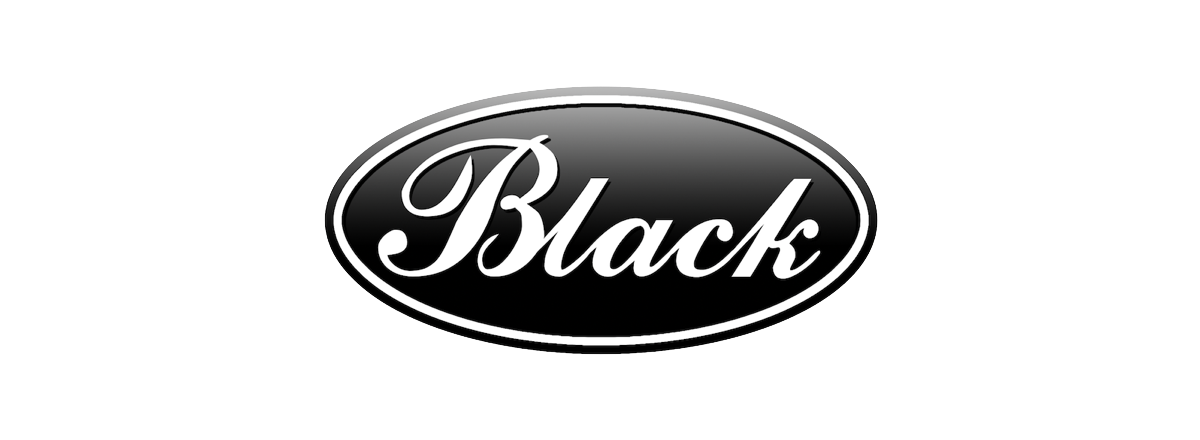
2 <h2 align="center">The Uncompromising Code Formatter</h2>
5 <a href="https://travis-ci.org/ambv/black"><img alt="Build Status" src="https://travis-ci.org/ambv/black.svg?branch=master"></a>
6 <a href="http://black.readthedocs.io/en/latest/?badge=latest"><img alt="Documentation Status" src="http://readthedocs.org/projects/black/badge/?version=latest"></a>
7 <a href="https://coveralls.io/github/ambv/black?branch=master"><img alt="Coverage Status" src="https://coveralls.io/repos/github/ambv/black/badge.svg?branch=master"></a>
8 <a href="https://github.com/ambv/black/blob/master/LICENSE"><img alt="License: MIT" src="http://black.readthedocs.io/en/latest/_static/license.svg"></a>
9 <a href="https://pypi.python.org/pypi/black"><img alt="PyPI" src="http://black.readthedocs.io/en/latest/_static/pypi.svg"></a>
10 <a href="https://github.com/ambv/black"><img alt="Code style: black" src="https://img.shields.io/badge/code%20style-black-000000.svg"></a>
13 > “Any color you like.”
16 *Black* is the uncompromising Python code formatter. By using it, you
17 agree to cease control over minutiae of hand-formatting. In return,
18 *Black* gives you speed, determinism, and freedom from `pycodestyle`
19 nagging about formatting. You will save time and mental energy for
20 more important matters.
22 Blackened code looks the same regardless of the project you're reading.
23 Formatting becomes transparent after a while and you can focus on the
26 *Black* makes code review faster by producing the smallest diffs
30 ## Installation and Usage
34 *Black* can be installed by running `pip install black`. It requires
35 Python 3.6.0+ to run but you can reformat Python 2 code with it, too.
40 To get started right away with sensible defaults:
43 black {source_file_or_directory}
46 ### Command line options
48 Black doesn't provide many options. You can list them by running
52 black [OPTIONS] [SRC]...
55 -l, --line-length INTEGER Where to wrap around. [default: 88]
56 --check Don't write the files back, just return the
57 status. Return code 0 means nothing would
58 change. Return code 1 means some files would be
59 reformatted. Return code 123 means there was an
61 --diff Don't write the files back, just output a diff
62 for each file on stdout.
63 --fast / --safe If --fast given, skip temporary sanity checks.
65 -q, --quiet Don't emit non-error messages to stderr. Errors
66 are still emitted, silence those with
68 --version Show the version and exit.
69 --help Show this message and exit.
72 *Black* is a well-behaved Unix-style command-line tool:
73 * it does nothing if no sources are passed to it;
74 * it will read from standard input and write to standard output if `-`
75 is used as the filename;
76 * it only outputs messages to users on standard error;
77 * exits with code 0 unless an internal error occured (or `--check` was
81 ### NOTE: This is an early pre-release
83 *Black* can already successfully format itself and the standard library.
84 It also sports a decent test suite. However, it is still very new.
85 Things will probably be wonky for a while. This is made explicit by the
86 "Alpha" trove classifier, as well as by the "a" in the version number.
87 What this means for you is that **until the formatter becomes stable,
88 you should expect some formatting to change in the future**.
90 Also, as a temporary safety measure, *Black* will check that the
91 reformatted code still produces a valid AST that is equivalent to the
92 original. This slows it down. If you're feeling confident, use
96 ## The *Black* code style
98 *Black* reformats entire files in place. It is not configurable. It
99 doesn't take previous formatting into account. It doesn't reformat
100 blocks that start with `# fmt: off` and end with `# fmt: on`. It also
101 recognizes [YAPF](https://github.com/google/yapf)'s block comments to
102 the same effect, as a courtesy for straddling code.
105 ### How *Black* wraps lines
107 *Black* ignores previous formatting and applies uniform horizontal
108 and vertical whitespace to your code. The rules for horizontal
109 whitespace are pretty obvious and can be summarized as: do whatever
110 makes `pycodestyle` happy. The coding style used by *Black* can be
111 viewed as a strict subset of PEP 8.
113 As for vertical whitespace, *Black* tries to render one full expression
114 or simple statement per line. If this fits the allotted line length,
129 If not, *Black* will look at the contents of the first outer matching
130 brackets and put that in a separate indented line.
134 l = [[n for n in list_bosses()], [n for n in list_employees()]]
139 [n for n in list_bosses()], [n for n in list_employees()]
143 If that still doesn't fit the bill, it will decompose the internal
144 expression further using the same rule, indenting matching brackets
145 every time. If the contents of the matching brackets pair are
146 comma-separated (like an argument list, or a dict literal, and so on)
147 then *Black* will first try to keep them on the same line with the
148 matching brackets. If that doesn't work, it will put all of them in
153 def very_important_function(template: str, *variables, file: os.PathLike, debug: bool = False):
154 """Applies `variables` to the `template` and writes to `file`."""
155 with open(file, 'w') as f:
160 def very_important_function(
166 """Applies `variables` to the `template` and writes to `file`."""
167 with open(file, "w") as f:
171 You might have noticed that closing brackets are always dedented and
172 that a trailing comma is always added. Such formatting produces smaller
173 diffs; when you add or remove an element, it's always just one line.
174 Also, having the closing bracket dedented provides a clear delimiter
175 between two distinct sections of the code that otherwise share the same
176 indentation level (like the arguments list and the docstring in the
182 You probably noticed the peculiar default line length. *Black* defaults
183 to 88 characters per line, which happens to be 10% over 80. This number
184 was found to produce significantly shorter files than sticking with 80
185 (the most popular), or even 79 (used by the standard library). In
186 general, [90-ish seems like the wise choice](https://youtu.be/wf-BqAjZb8M?t=260).
188 If you're paid by the line of code you write, you can pass
189 `--line-length` with a lower number. *Black* will try to respect that.
190 However, sometimes it won't be able to without breaking other rules. In
191 those rare cases, auto-formatted code will exceed your allotted limit.
193 You can also increase it, but remember that people with sight disabilities
194 find it harder to work with line lengths exceeding 100 characters.
195 It also adversely affects side-by-side diff review on typical screen
196 resolutions. Long lines also make it harder to present code neatly
197 in documentation or talk slides.
199 If you're using Flake8, you can bump `max-line-length` to 88 and forget
200 about it. Alternatively, use [Bugbear](https://github.com/PyCQA/flake8-bugbear)'s
201 B950 warning instead of E501 and keep the max line length at 80 which
202 you are probably already using. You'd do it like this:
207 select = C,E,F,W,B,B950
211 You'll find *Black*'s own .flake8 config file is configured like this.
212 If you're curious about the reasoning behind B950, Bugbear's documentation
213 explains it. The tl;dr is "it's like highway speed limits, we won't
214 bother you if you overdo it by a few km/h".
219 *Black* avoids spurious vertical whitespace. This is in the spirit of
220 PEP 8 which says that in-function vertical whitespace should only be
221 used sparingly. One exception is control flow statements: *Black* will
222 always emit an extra empty line after ``return``, ``raise``, ``break``,
223 ``continue``, and ``yield``. This is to make changes in control flow
224 more prominent to readers of your code.
226 *Black* will allow single empty lines inside functions, and single and
227 double empty lines on module level left by the original editors, except
228 when they're within parenthesized expressions. Since such expressions
229 are always reformatted to fit minimal space, this whitespace is lost.
231 It will also insert proper spacing before and after function definitions.
232 It's one line before and after inner functions and two lines before and
233 after module-level functions. *Black* will put those empty lines also
234 between the function definition and any standalone comments that
235 immediately precede the given function. If you want to comment on the
236 entire function, use a docstring or put a leading comment in the function
242 *Black* will add trailing commas to expressions that are split
243 by comma where each element is on its own line. This includes function
246 Unnecessary trailing commas are removed if an expression fits in one
247 line. This makes it 1% more likely that your line won't exceed the
248 allotted line length limit. Moreover, in this scenario, if you added
249 another argument to your call, you'd probably fit it in the same line
250 anyway. That doesn't make diffs any larger.
252 One exception to removing trailing commas is tuple expressions with
253 just one element. In this case *Black* won't touch the single trailing
254 comma as this would unexpectedly change the underlying data type. Note
255 that this is also the case when commas are used while indexing. This is
256 a tuple in disguise: ```numpy_array[3, ]```.
258 One exception to adding trailing commas is function signatures
259 containing `*`, `*args`, or `**kwargs`. In this case a trailing comma
260 is only safe to use on Python 3.6. *Black* will detect if your file is
261 already 3.6+ only and use trailing commas in this situation. If you
262 wonder how it knows, it looks for f-strings and existing use of trailing
263 commas in function signatures that have stars in them. In other words,
264 if you'd like a trailing comma in this situation and *Black* didn't
265 recognize it was safe to do so, put it there manually and *Black* will
270 *Black* prefers double quotes (`"` and `"""`) over single quotes (`'`
271 and `'''`). It will replace the latter with the former as long as it
272 does not result in more backslash escapes than before.
274 The main reason to standardize on a single form of quotes is aesthetics.
275 Having one kind of quotes everywhere reduces reader distraction.
276 It will also enable a future version of *Black* to merge consecutive
277 string literals that ended up on the same line (see
278 [#26](https://github.com/ambv/black/issues/26) for details).
280 Why settle on double quotes? They anticipate apostrophes in English
281 text. They match the docstring standard described in PEP 257. An
282 empty string in double quotes (`""`) is impossible to confuse with
283 a one double-quote regardless of fonts and syntax highlighting used.
284 On top of this, double quotes for strings are consistent with C which
285 Python interacts a lot with.
287 On certain keyboard layouts like US English, typing single quotes is
288 a bit easier than double quotes. The latter requires use of the Shift
289 key. My recommendation here is to keep using whatever is faster to type
290 and let *Black* handle the transformation.
292 ### Line Breaks & Binary Operators
294 *Black* will break a line before a binary operator when splitting a block
295 of code over multiple lines. This is so that *Black* is compliant with the
296 recent changes in the [PEP 8](https://www.python.org/dev/peps/pep-0008/#should-a-line-break-before-or-after-a-binary-operator)
297 style guide, which emphasizes that this approach improves readability.
299 This behaviour may raise ``W503 line break before binary operator`` warnings in
300 style guide enforcement tools like Flake8. Since ``W503`` is not PEP 8 compliant,
301 you should tell Flake8 to ignore these warnings.
304 ## Editor integration
308 Use [proofit404/blacken](https://github.com/proofit404/blacken).
317 2. Locate your `black` installation folder.
319 $ which black # MacOS/Linux
320 /usr/local/bin/black # possible location
324 $ where black # Windows
325 %LocalAppData%\Programs\Python\Python36-32\Scripts\black.exe # possible location
327 3. Open External tools in PyCharm with `File -> Settings -> Tools -> External Tools`.
329 4. Click the + icon to add a new external tool with the following values:
331 - Description: Black is the uncompromising Python code formatter.
332 - Program: <install_location_from_step_2>
333 - Arguments: $FilePath$
335 5. Format the currently opened file by selecting `Tools -> External Tools -> black`.
336 - Alternatively, you can set a keyboard shortcut by navigating to `Preferences -> Keymap`.
341 Commands and shortcuts:
343 * `,=` or `:Black` to format the entire file (ranges not supported);
344 * `:BlackUpgrade` to upgrade *Black* inside the virtualenv;
345 * `:BlackVersion` to get the current version of *Black* inside the
349 * `g:black_fast` (defaults to `0`)
350 * `g:black_linelength` (defaults to `88`)
351 * `g:black_virtualenv` (defaults to `~/.vim/black`)
353 To install, copy the plugin from [vim/plugin/black.vim](https://github.com/ambv/black/tree/master/vim/plugin/black.vim).
354 Let me know if this requires any changes to work with Vim 8's builtin
355 `packadd`, or Pathogen, or Vundle, and so on.
357 This plugin **requires Vim 7.0+ built with Python 3.6+ support**. It
358 needs Python 3.6 to be able to run *Black* inside the Vim process which
359 is much faster than calling an external command.
361 On first run, the plugin creates its own virtualenv using the right
362 Python version and automatically installs *Black*. You can upgrade it later
363 by calling `:BlackUpgrade` and restarting Vim.
365 If you need to do anything special to make your virtualenv work and
366 install *Black* (for example you want to run a version from master), just
367 create a virtualenv manually and point `g:black_virtualenv` to it.
368 The plugin will use it.
370 **How to get Vim with Python 3.6?**
371 On Ubuntu 17.10 Vim comes with Python 3.6 by default.
372 On macOS with HomeBrew run: `brew install vim --with-python3`.
373 When building Vim from source, use:
374 `./configure --enable-python3interp=yes`. There's many guides online how
378 ### Visual Studio Code
380 Use [joslarson.black-vscode](https://marketplace.visualstudio.com/items?itemName=joslarson.black-vscode).
385 Atom/Nuclide integration is planned by the author, others will
386 require external contributions.
388 Patches welcome! ✨ 🍰 ✨
390 Any tool that can pipe code through *Black* using its stdio mode (just
391 [use `-` as the file name](http://www.tldp.org/LDP/abs/html/special-chars.html#DASHREF2)).
392 The formatted code will be returned on stdout (unless `--check` was
393 passed). *Black* will still emit messages on stderr but that shouldn't
394 affect your use case.
396 This can be used for example with PyCharm's [File Watchers](https://www.jetbrains.com/help/pycharm/file-watchers.html).
399 ## Version control integration
401 Use [pre-commit](https://pre-commit.com/). Once you [have it
402 installed](https://pre-commit.com/#install), add this to the
403 `.pre-commit-config.yaml` in your repository:
406 - repo: https://github.com/ambv/black
410 args: [--line-length=88, --safe]
411 python_version: python3.6
413 Then run `pre-commit install` and you're ready to go.
415 `args` in the above config is optional but shows you how you can change
416 the line length if you really need to. If you're already using Python
417 3.7, switch the `python_version` accordingly. Finally, `stable` is a tag
418 that is pinned to the latest release on PyPI. If you'd rather run on
419 master, this is also an option.
423 **Dusty Phillips**, [writer](https://smile.amazon.com/s/ref=nb_sb_noss?url=search-alias%3Daps&field-keywords=dusty+phillips):
425 > Black is opinionated so you don't have to be.
427 **Hynek Schlawack**, [creator of `attrs`](http://www.attrs.org/), core
428 developer of Twisted and CPython:
430 > An auto-formatter that doesn't suck is all I want for Xmas!
432 **Carl Meyer**, [Django](https://www.djangoproject.com/) core developer:
434 > At least the name is good.
436 **Kenneth Reitz**, creator of [`requests`](http://python-requests.org/)
437 and [`pipenv`](https://docs.pipenv.org/):
439 > This vastly improves the formatting of our code. Thanks a ton!
444 Use the badge in your project's README.md:
447 [](https://github.com/ambv/black)
450 Looks like this: [](https://github.com/ambv/black)
458 ## Contributing to Black
460 In terms of inspiration, *Black* is about as configurable as *gofmt* and
461 *rustfmt* are. This is deliberate.
463 Bug reports and fixes are always welcome! However, before you suggest a
464 new feature or configuration knob, ask yourself why you want it. If it
465 enables better integration with some workflow, fixes an inconsistency,
466 speeds things up, and so on - go for it! On the other hand, if your
467 answer is "because I don't like a particular formatting" then you're not
468 ready to embrace *Black* yet. Such changes are unlikely to get accepted.
469 You can still try but prepare to be disappointed.
471 More details can be found in [CONTRIBUTING](CONTRIBUTING.md).
478 * added `--quiet` (#78)
480 * added [pre-commit](https://pre-commit.com) integration (#103, #104)
482 * fixed reporting on `--check` with multiple files (#101, #102)
484 * fixed removing backslash escapes from raw strings (#100, #105)
488 * added `--diff` (#87)
490 * add line breaks before all delimiters, except in cases like commas, to
491 better comply with PEP 8 (#73)
493 * standardize string literals to use double quotes (almost) everywhere
496 * fixed handling of standalone comments within nested bracketed
497 expressions; Black will no longer produce super long lines or put all
498 standalone comments at the end of the expression (#22)
500 * fixed 18.3a4 regression: don't crash and burn on empty lines with
501 trailing whitespace (#80)
503 * fixed 18.3a4 regression: `# yapf: disable` usage as trailing comment
504 would cause Black to not emit the rest of the file (#95)
506 * when CTRL+C is pressed while formatting many files, Black no longer
507 freaks out with a flurry of asyncio-related exceptions
509 * only allow up to two empty lines on module level and only single empty
510 lines within functions (#74)
515 * `# fmt: off` and `# fmt: on` are implemented (#5)
517 * automatic detection of deprecated Python 2 forms of print statements
518 and exec statements in the formatted file (#49)
520 * use proper spaces for complex expressions in default values of typed
521 function arguments (#60)
523 * only return exit code 1 when --check is used (#50)
525 * don't remove single trailing commas from square bracket indexing
528 * don't omit whitespace if the previous factor leaf wasn't a math
531 * omit extra space in kwarg unpacking if it's the first argument (#46)
533 * omit extra space in [Sphinx auto-attribute comments](http://www.sphinx-doc.org/en/stable/ext/autodoc.html#directive-autoattribute)
539 * don't remove single empty lines outside of bracketed expressions
542 * added ability to pipe formatting from stdin to stdin (#25)
544 * restored ability to format code with legacy usage of `async` as
547 * even better handling of numpy-style array indexing (#33, again)
552 * changed positioning of binary operators to occur at beginning of lines
553 instead of at the end, following [a recent change to PEP8](https://github.com/python/peps/commit/c59c4376ad233a62ca4b3a6060c81368bd21e85b)
556 * ignore empty bracket pairs while splitting. This avoids very weirdly
557 looking formattings (#34, #35)
559 * remove a trailing comma if there is a single argument to a call
561 * if top level functions were separated by a comment, don't put four
562 empty lines after the upper function
564 * fixed unstable formatting of newlines with imports
566 * fixed unintentional folding of post scriptum standalone comments
567 into last statement if it was a simple statement (#18, #28)
569 * fixed missing space in numpy-style array indexing (#33)
571 * fixed spurious space after star-based unary expressions (#31)
578 * only put trailing commas in function signatures and calls if it's
579 safe to do so. If the file is Python 3.6+ it's always safe, otherwise
580 only safe if there are no `*args` or `**kwargs` used in the signature
583 * fixed invalid spacing of dots in relative imports (#6, #13)
585 * fixed invalid splitting after comma on unpacked variables in for-loops
588 * fixed spurious space in parenthesized set expressions (#7)
590 * fixed spurious space after opening parentheses and in default
593 * fixed spurious space after unary operators when the operand was
594 a complex expression (#15)
599 * first published version, Happy 🍰 Day 2018!
603 * date-versioned (see: https://calver.org/)
608 Glued together by [Łukasz Langa](mailto:lukasz@langa.pl).
610 Maintained with [Carol Willing](mailto:carolcode@willingconsulting.com),
611 [Carl Meyer](mailto:carl@oddbird.net), and
612 [Mika Naylor](mailto:mail@autophagy.io).
614 Multiple contributions by:
615 * [Anthony Sottile](mailto:asottile@umich.edu)
616 * [Artem Malyshev](mailto:proofit404@gmail.com)
617 * [Daniel M. Capella](mailto:polycitizen@gmail.com)
618 * [Eli Treuherz](mailto:eli.treuherz@cgi.com)
620 * [Ivan Katanić](mailto:ivan.katanic@gmail.com)
621 * [Osaetin Daniel](mailto:osaetindaniel@gmail.com)
622 * [Zsolt Dollenstein](mailto:zsol.zsol@gmail.com)